[LeetCode/Kotlin]Easy - 118. Pascal's Triangle
2023. 5. 29. 19:13ㆍLeetCode/Kotlin | Easy
728x90
반응형
Pascal's Triangle - LeetCode
Can you solve this real interview question? Pascal's Triangle - Given an integer numRows, return the first numRows of Pascal's triangle. In Pascal's triangle, each number is the sum of the two numbers directly above it as shown: [https://upload.wikimedia.o
leetcode.com
문제
Given an integer numRows, return the first numRows of Pascal's triangle.
In Pascal's triangle, each number is the sum of the two numbers directly above it as shown:
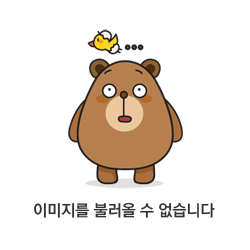
Example 1:
Input: numRows = 5
Output: [[1],[1,1],[1,2,1],[1,3,3,1],[1,4,6,4,1]]
Example 2:
Input: numRows = 1
Output: [[1]]
풀이
나의 풀이법
풀이 접근 과정
그냥 앞에 List에서 값을 가져와서 계산하는 방식으로 하면 되겠구나 생각했다.
Kotlin에서는 List보다는 Array를 쓰는 편이 나에게 더 편하므로 Array를 List로 형변환시켰다.
최종 소스코드
class Solution {
fun generate(numRows: Int): List<List<Int>> {
val pascals = Array(numRows) { listOf<Int>() }
for (i in 0 until numRows) {
if (i == 0 || i == 1) pascals[i] = Array(i+1){1}.toList()
else {
val pas = Array(i+1) { 1 }
for (j in 1 until i) {
pas[j] = pascals[i-1][j-1] + pascals[i-1][j]
}
pascals[i] = pas.toList()
}
}
return pascals.toList()
}
}
- 파스칼 배열을 담을 pascals를 선언한다.
- 나중에 Array를 List로 변환할 것이기 때문에, pascals는 최초 선언할 때 Array<List<Int>> 형태이다.
- index를 0부터 입력받은 numRows까지 돌린다.
- index가 0 혹은 1인 경우, 해당 index의 +1 크기만큼 Array를 만들고 모두 1로 초기화한다.
- 그리고 List로 변환하여 pascals[index]에 List를 넣는다.
- index가 2 이상인 경우, index+1 크기의Array를 만들고 처음은 모두 1로 초기화한다.후에 Array를 List로 변환하여 pascals[index]에 List를 넣는다.
- 그리고 pascals의 [index-1] 위치에서 두값을 가져와 더하여 Array에 넣는다.
- 그렇게 만들어진pascals를 List로 변환하여 리턴한다.
728x90
반응형
'LeetCode > Kotlin | Easy' 카테고리의 다른 글
[LeetCode/Kotlin]Easy - 1470. Shuffle the Array (0) | 2023.06.05 |
---|---|
[LeetCode/Kotlin]Easy - 14. Longest Common Prefix (0) | 2023.05.29 |
[LeetCode/Kotlin]Easy - 938. Range Sum of BST (0) | 2023.05.23 |
[LeetCode/Kotlin]Easy - 70. Climbing Stairs (0) | 2023.05.23 |
[LeetCode/Kotlin]Easy - 2016. Maximum Difference Between Increasing Elements (0) | 2023.05.16 |